How to Find All Files with a specific Extension with Ansible?
I’m going to show you a live Playbook with some simple Ansible code. I’m Luca Berton and welcome to today’s episode of Ansible Pilot.
Ansible Find All Files with Extension
ansible.builtin.find
- Return a list of files based on specific criteria
Today we’re talking about the Ansible module find
.
The full name is ansible.builtin.find
, which means that is part of the collection included in the ansible-core
builtin collection.
This module returns a list of files based on specific criteria using the find
popular Unix command.
Parameters
- paths string - List of paths of directories to search
- hidden boolean - no/yes
- recurse boolean - recursively descend into the directory looking for files
- file_type string - file/directory/any/link
- patterns list - search (shell or regex) pattern(s)
- use_regex boolean - no/yes - file globs (shell) / python regexes
The most important parameters of the find
module for this use case.
The mandatory parameter paths
specify the list of paths of directories to search.
You could include hidden files with the hidden
parameter.
As well as recurse in any directory under the main path with the recurse
parameter.
Another useful parameter is file_type
, which defaults to file
but you could filter for directory
, link
, or any
filesystem object type.
Specify what to search under the patterns
list.
Ansible by default uses file globs (shell) patterns but you could specify also python regexes enabling the use_regex
parameter.
Links
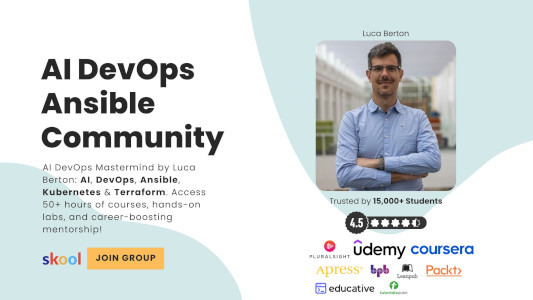
Playbook
How to Find All Files with Extension with Ansible Playbook. I’m going to search only the files and directories under the example folder of my login users (devops) and list all the files with the “.cnf” extension. This code has no dangerous effect on the target machine.
code
---
- name: find Playbook
hosts: all
vars:
mypath: "/home/devops/example"
mypattern: '*.cnf'
tasks:
- name: search files
ansible.builtin.find:
paths: "{{ mypath }}"
hidden: true
recurse: true
file_type: any
patterns: "{{ mypattern }}"
register: found_files
- name: print files
ansible.builtin.debug:
var: found_files
execution
$ ansible-playbook -i virtualmachines/demo/inventory file_management/file_find.yml
PLAY [find Playbook] **********************************************************************************
TASK [Gathering Facts] ****************************************************************************
ok: [demo.example.com]
TASK [search files] *******************************************************************************
ok: [demo.example.com]
TASK [print files] ********************************************************************************
ok: [demo.example.com] => {
"found_files": {
"changed": false,
"examined": 3,
"failed": false,
"files": [
{
"atime": 1657183703.9653573,
"ctime": 1657183703.9653573,
"dev": 64768,
"gid": 10,
"gr_name": "wheel",
"inode": 134965918,
"isblk": false,
"ischr": false,
"isdir": false,
"isfifo": false,
"isgid": false,
"islnk": false,
"isreg": true,
"issock": false,
"isuid": false,
"mode": "0644",
"mtime": 1657183703.9653573,
"nlink": 1,
"path": "/home/devops/example/file1.cnf",
"pw_name": "devops",
"rgrp": true,
"roth": true,
"rusr": true,
"size": 0,
"uid": 1001,
"wgrp": false,
"woth": false,
"wusr": true,
"xgrp": false,
"xoth": false,
"xusr": false
},
{
"atime": 1657183705.7742639,
"ctime": 1657183705.7742639,
"dev": 64768,
"gid": 10,
"gr_name": "wheel",
"inode": 134965931,
"isblk": false,
"ischr": false,
"isdir": false,
"isfifo": false,
"isgid": false,
"islnk": false,
"isreg": true,
"issock": false,
"isuid": false,
"mode": "0644",
"mtime": 1657183705.7742639,
"nlink": 1,
"path": "/home/devops/example/file2.cnf",
"pw_name": "devops",
"rgrp": true,
"roth": true,
"rusr": true,
"size": 0,
"uid": 1001,
"wgrp": false,
"woth": false,
"wusr": true,
"xgrp": false,
"xoth": false,
"xusr": false
}
],
"matched": 2,
"msg": "All paths examined",
"skipped_paths": {}
}
}
PLAY RECAP ****************************************************************************************
demo.example.com : ok=3 changed=0 unreachable=0 failed=0 skipped=0 rescued=0 ignored=0
idempotency
$ ansible-playbook -i virtualmachines/demo/inventory file_management/file_find.yml
PLAY [find Playbook] **********************************************************************************
TASK [Gathering Facts] ****************************************************************************
ok: [demo.example.com]
TASK [search files] *******************************************************************************
ok: [demo.example.com]
TASK [print files] ********************************************************************************
ok: [demo.example.com] => {
"found_files": {
"changed": false,
"examined": 3,
"failed": false,
"files": [
{
"atime": 1657183703.9653573,
"ctime": 1657183703.9653573,
"dev": 64768,
"gid": 10,
"gr_name": "wheel",
"inode": 134965918,
"isblk": false,
"ischr": false,
"isdir": false,
"isfifo": false,
"isgid": false,
"islnk": false,
"isreg": true,
"issock": false,
"isuid": false,
"mode": "0644",
"mtime": 1657183703.9653573,
"nlink": 1,
"path": "/home/devops/example/file1.cnf",
"pw_name": "devops",
"rgrp": true,
"roth": true,
"rusr": true,
"size": 0,
"uid": 1001,
"wgrp": false,
"woth": false,
"wusr": true,
"xgrp": false,
"xoth": false,
"xusr": false
},
{
"atime": 1657183705.7742639,
"ctime": 1657183705.7742639,
"dev": 64768,
"gid": 10,
"gr_name": "wheel",
"inode": 134965931,
"isblk": false,
"ischr": false,
"isdir": false,
"isfifo": false,
"isgid": false,
"islnk": false,
"isreg": true,
"issock": false,
"isuid": false,
"mode": "0644",
"mtime": 1657183705.7742639,
"nlink": 1,
"path": "/home/devops/example/file2.cnf",
"pw_name": "devops",
"rgrp": true,
"roth": true,
"rusr": true,
"size": 0,
"uid": 1001,
"wgrp": false,
"woth": false,
"wusr": true,
"xgrp": false,
"xoth": false,
"xusr": false
}
],
"matched": 2,
"msg": "All paths examined",
"skipped_paths": {}
}
}
PLAY RECAP ****************************************************************************************
demo.example.com : ok=3 changed=0 unreachable=0 failed=0 skipped=0 rescued=0 ignored=0
before execution
$ ssh [email protected]
[devops@demo ~]$ cd example
[devops@demo example]$ ls
file1.cnf file2.cnf file3.txt
[devops@demo example]$ tree
.
|-- file1.cnf
|-- file2.cnf
`-- file3.txt
0 directories, 3 files
[devops@demo example]$
after execution
$ ssh [email protected]
[devops@demo ~]$ cd example
[devops@demo example]$ ls
file1.cnf file2.cnf file3.txt
[devops@demo example]$ tree
.
|-- file1.cnf
|-- file2.cnf
`-- file3.txt
0 directories, 3 files
[devops@demo example]$
Conclusion
Now you know how to Find All Files with Extension with Ansible. Subscribe to the YouTube channel, Medium, and Website, X (formerly Twitter) to not miss the next episode of the Ansible Pilot.
Academy
Learn the Ansible automation technology with some real-life examples in my
Udemy 300+ Lessons Video Course.
My book Ansible By Examples: 200+ Automation Examples For Linux and Windows System Administrator and DevOps
Donate
Want to keep this project going? Please donate