Customizing Ansible: Ansible Module Creation
Unlocking Ansible’s Potential Through Custom Python Modules.
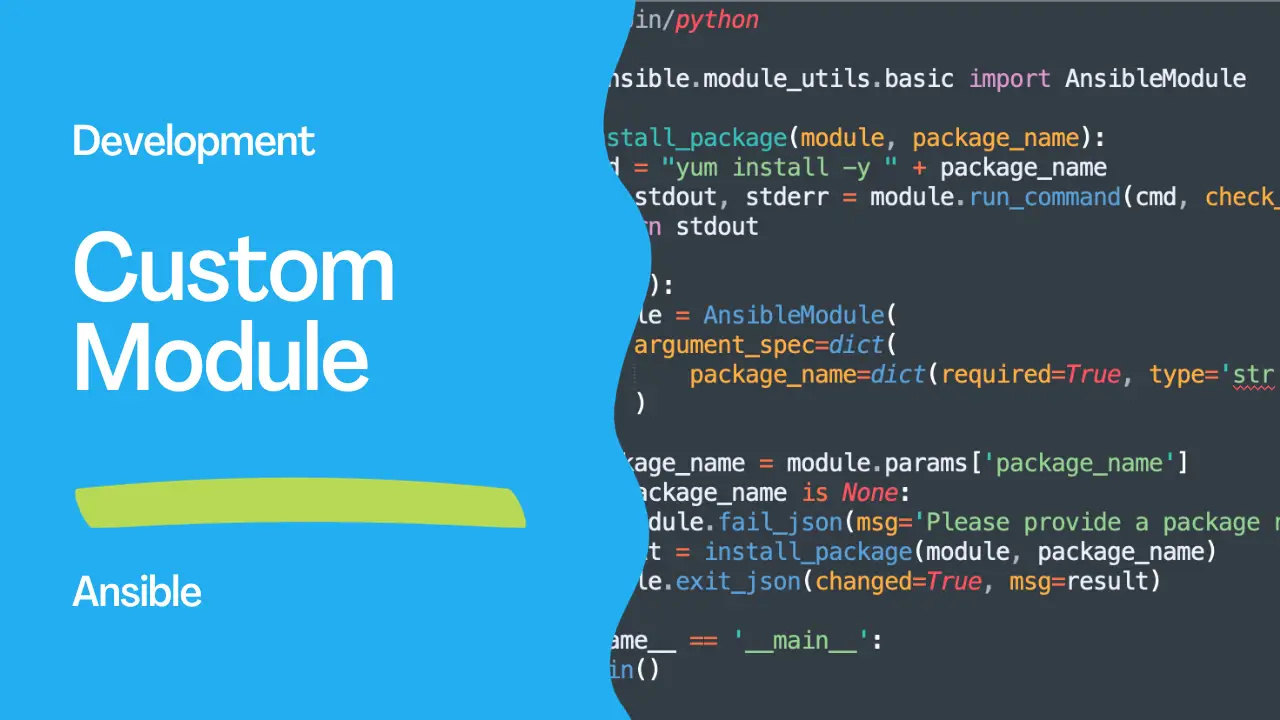
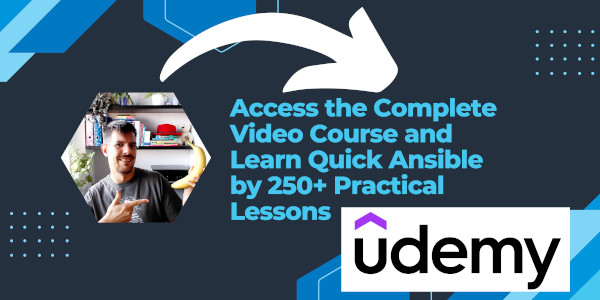
***Original author: Nikhil Kumar in Customizing Ansible: Ansible Module Creation ***
Introduction
Ansible is a powerful open-source software used for configuration management, provisioning, and application deployment. It belongs to the realm of Infrastructure as Code (IaC), where infrastructure is defined and managed through code. Ansible enables you to create and deploy infrastructure on various platforms, including cloud services like AWS and Azure, as well as hypervisors.
One of the key components that makes Ansible so versatile and extensible is the concept of Ansible Modules. These modules are reusable, standalone units of code designed to perform specific tasks on managed or target nodes. While Ansible modules can be written in any language capable of producing JSON output, Python is the most popular and recommended choice. This is because Ansible itself is written in Python, which makes it seamlessly integrate with JSON data.
In this article, we will explore the steps involved in creating custom Ansible modules using Python. We’ll also provide an example to illustrate the process.
Creating Custom Ansible Modules in Python
To create a custom Ansible module in Python, follow these steps:
- Set Up Your Environment: Begin by creating a library directory in your working environment where you will store your custom modules. This directory will contain the Python files for your modules. Let’s call it library.
- Create Your Module File: Inside the library directory, create your custom module file, e.g., custom_module.py. This is where you’ll write the code for your module.
- Utilize Ansible Module Utils: Ansible provides a module_utils library that can be used for creating custom modules. Import this library in your module to access the AnsibleModule class, which is used for handling module arguments, inputs, outputs, and errors.
- Define Module Inputs: Define the module inputs that you want to take from the users. This can include parameters like username, package_name, or any other input required for your module.
- Write Module Logic: Within your module file, write the logic for the module. This logic should specify what actions the module will perform on the target node and how it will return the output.
- Testing: Test your module rigorously with various test cases to ensure it functions as expected. Provide different sets of inputs and verify whether the module produces the correct output.
Example: Installing Packages on Linux with a Custom Module
Here’s an example of a custom Ansible module written in Python. This module installs packages on a Linux system using the yum package manager:
#!/usr/bin/python
from ansible.module_utils.basic import AnsibleModule
def install_package(module, package_name):
cmd = "yum install -y " + package_name
rc, stdout, stderr = module.run_command(cmd, check_rc=True)
return stdout
def main():
module = AnsibleModule(
argument_spec=dict(
package_name=dict(required=True, type='str'),
)
)
package_name = module.params['package_name']
if package_name is None:
module.fail_json(msg='Please provide a package name')
result = install_package(module, package_name)
module.exit_json(changed=True, msg=result)
if __name__ == '__main__':
main()
In this example:
- We import the
ansible.module_utils
library to access the AnsibleModule class. - The
install_package
function takes the module object and the package name as arguments and uses the run_command method to execute the yum command to install the specified package. - In the
main
function, we create an AnsibleModule object with the required input parameters, and the install_package function is called with the package name. - We use
fail_json
to handle module failures, and in this case, we check whether the package name is provided; if not, it throws an error in JSON format and exits the module. - Finally, we use
exit_json
to return the result of the module execution. To use this custom module to install thehttpd
package, you can include the following task in your playbook:
---
- name: Use the Custom Module
hosts: localhost
tasks:
- name: install httpd
custom_module:
package_name: httpd
The Best Resources For Ansible
Certifications
Coursera Pro - Unlimited access to 7,000+ world-class courses, hands-on projects, and job-ready certificate programs—all included in your subscription
Video Course
Printed Book
Ansible For VMware by Examples
Ansible for Kubernetes by Example
Hands-on Ansible Automation
Red Hat Ansible Automation Platform
eBooks
Ansible by Examples: 200+ Automation Examples For Linux and Windows System Administrator and DevOps
Ansible Cookbook: A Comprehensive Guide to Unleashing the Power of Ansible via Best Practices, Troubleshooting, and Linting Rules with Luca Berton
Terraform By Example: A Practical Approach for Beginners to Learn Cloud Infrastructure with Terraform
Ansible For Windows By Examples: 50+ Automation Examples For Windows System Administrator And DevOps
Ansible For Linux by Examples: 100+ Automation Examples For Linux System Administrator and DevOps
Ansible Linux Filesystem By Examples: 40+ Automation Examples on Linux File and Directory Operation for Modern IT Infrastructure
Ansible For Security by Examples: 100+ Automation Examples to Automate Security and Verify Compliance for IT Modern Infrastructure
Ansible Tips and Tricks: 10+ Ansible Examples to Save Time and Automate More Tasks
Ansible Linux Users & Groups By Examples: 20+ Automation Examples on Linux Users and Groups Operation for Modern IT Infrastructure
Ansible For PostgreSQL by Examples: 10+ Examples To Automate Your PostgreSQL database
Ansible For Amazon Web Services AWS By Examples: 10+ Examples To Automate Your AWS Modern Infrastructure
Ansible Automation Platform By Example: A step-by-step guide for the most common user scenarios
Best Practices for Custom Ansible Module Creation
When creating custom Ansible modules, it’s essential to follow best practices to ensure the modules are effective and maintainable:
- Utilize Ansible Module Utils: Use the ansible.module_utils library to leverage the AnsibleModule class and streamline module development.
- Clear and Concise Module Names: Choose module names that clearly indicate the module’s purpose. A well-named module is self-explanatory and easy to understand.
- Consistent and Well-Documented Input Format: Define input parameters in a consistent and well-documented manner. Users should easily understand what inputs are required.
- Error Handling: Ensure that your module handles errors gracefully. Error messages should be informative and help users diagnose and resolve issues.
- Thorough Testing: Thoroughly test your module with different scenarios to confirm its correctness and reliability.
Conclusion
Creating custom Ansible modules is a rewarding and powerful way to automate infrastructure management. It allows you to extend Ansible’s functionality and automate complex tasks. By following best practices for module creation, you can develop high-quality, user-friendly Ansible modules. This, in turn, enables you to harness the full potential of Ansible for automating your infrastructure.
In conclusion, custom Ansible modules empower you to tailor your automation solutions to your specific needs, and they also provide an opportunity to contribute to the open-source Ansible community. So, don’t hesitate to explore the creation of your own custom Ansible modules and share your experiences and insights with the broader community.
Subscribe to the YouTube channel, Medium, and Website, X (formerly Twitter) to not miss the next episode of the Ansible Pilot.Academy
Learn the Ansible automation technology with some real-life examples in my
My book Ansible By Examples: 200+ Automation Examples For Linux and Windows System Administrator and DevOps
Donate
Want to keep this project going? Please donate