Cleaning the Build Directory in Python
Streamline Your Python Development Workflow with setup.py Enhancements
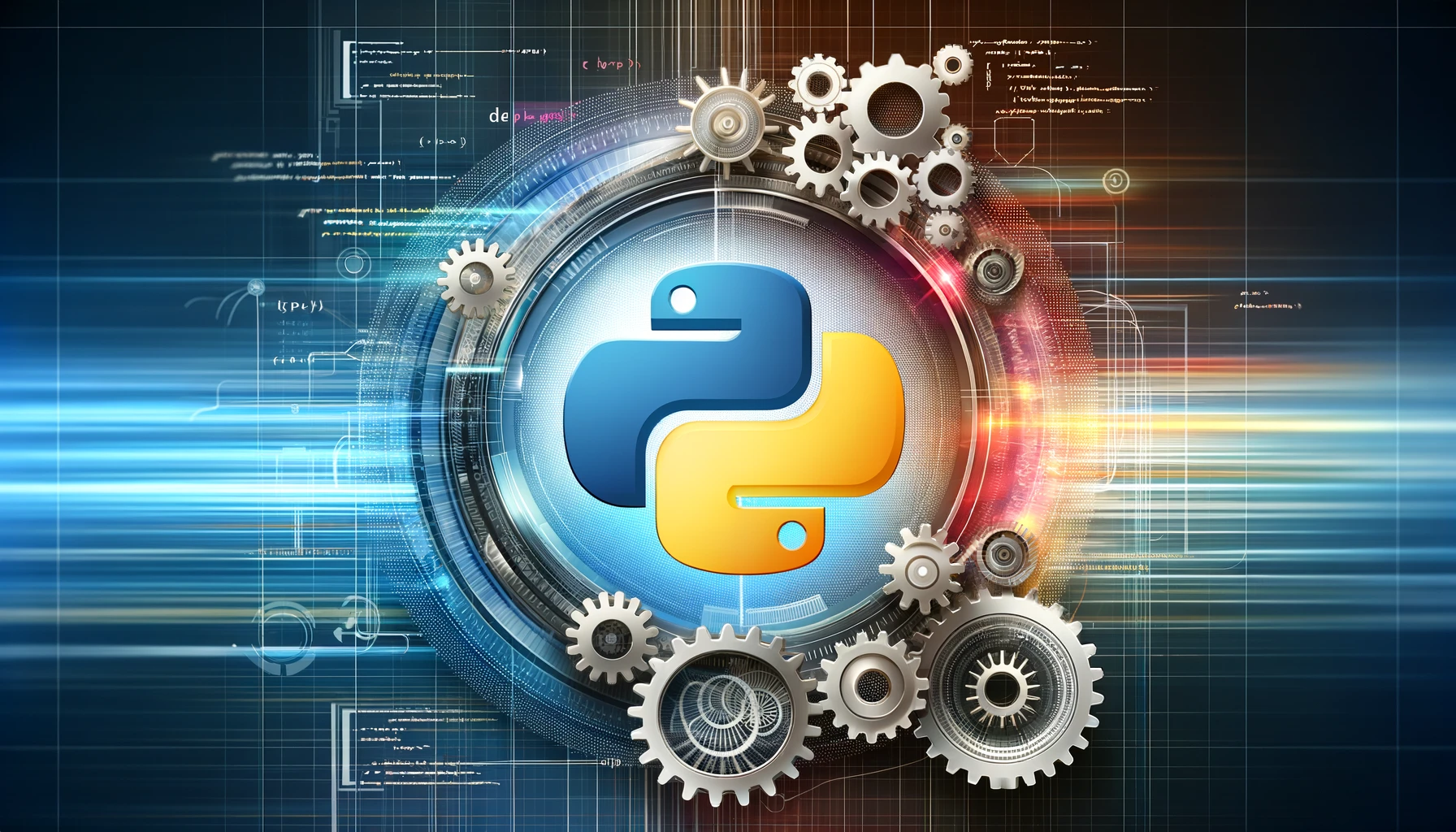
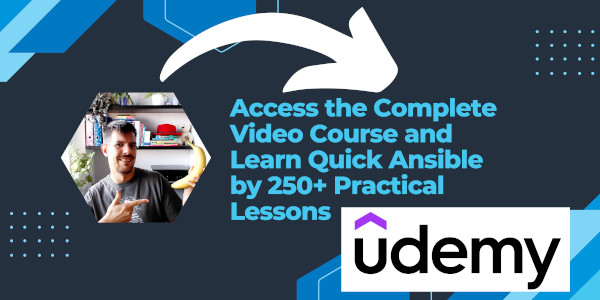
Introduction
In the dynamic world of Python development, maintaining a clean and efficient build environment is crucial for project stability and ease of updates. An often overlooked yet essential part of this process involves managing the build directory through your setup.py
script. This article delves into techniques for automating the pre-deletion and post-deletion of the build directory, ensuring a cleaner, more manageable project structure.
The Importance of a Clean Slate
The build directory in a Python project, typically generated during the build process, can become cluttered with outdated or unnecessary files over time. Cleaning this directory before and after each build can prevent potential conflicts and reduce the overall size of your project, making version control and distribution more straightforward.
Pre-Deletion and Post-Deletion Strategies
Pre-Deletion: To ensure a clean starting point, the build directory should be deleted before the setup process begins. This can be achieved programmatically by using distutils.dir_util.remove_tree
to remove the directory at the start of your setup.py
script.
Example:
from distutils.dir_util import remove_tree
# Remove the build directory at the start
remove_tree('build', verbose=True)
Post-Deletion: Cleaning up after your build is equally important. This step involves removing the build directory once the setup process has completed, ensuring that no unnecessary files linger in your project.
One effective method for post-deletion is to subclass specific setup commands, overriding their run
method to include a clean-up step at the end. This approach allows for targeted cleaning after critical operations, such as installing or building distributions.
Example:
from setuptools import setup, Command
class CustomInstall(Command):
def run(self):
# Your setup tasks here
super().run()
# Cleanup the build directory
remove_tree('build', verbose=True)
setup(
# Your setup config here
cmdclass={
'install': CustomInstall,
},
)
Utilizing the clean
Command
The clean
command is a built-in option in setuptools
that can be used to clear the build directory. Executing python setup.py clean --all
removes the build directory along with other temporary files created during the build process. This command can be incorporated into your development workflow to automate the cleaning process.
However, it’s worth noting that not all projects may support the clean
command out of the box. For projects where the clean
command is not effective, a custom script or command can be employed to ensure a thorough clean-up.
Advanced Cleaning Techniques
For a more comprehensive cleaning approach that addresses the build directory and other areas like dist
and egg-info
, consider implementing a custom cleaning script or extending the clean
command within your setup.py
. This ensures that all artifacts of the build process are removed, leaving a clean environment for the next build cycle.
Example:
from distutils.command.clean import clean as Clean
class MyClean(Clean):
def run(self):
# Custom cleaning logic here
super().run()
# Additional directories to clean
remove_tree('dist', verbose=True)
remove_tree('my_project.egg-info', verbose=True)
setup(
# Your setup config here
cmdclass={
'clean': MyClean,
},
)
Conclusion
Managing the build directory through your setup.py
script is a pivotal step in maintaining a clean and efficient development environment. By employing pre-deletion and post-deletion strategies, along with the built-in clean
command and custom cleaning scripts, you can ensure that your Python project remains streamlined and manageable. Remember, a clean project is the foundation of a stable and scalable application.
Academy
Learn the Ansible automation technology with some real-life examples in my
My book Ansible By Examples: 200+ Automation Examples For Linux and Windows System Administrator and DevOps
Donate
Want to keep this project going? Please donate