Automating YouTube Playlist Information Retrieval with Python’s Pytube Library
Automating YouTube Playlist Analysis and Information Retrieval with Python and Pytube
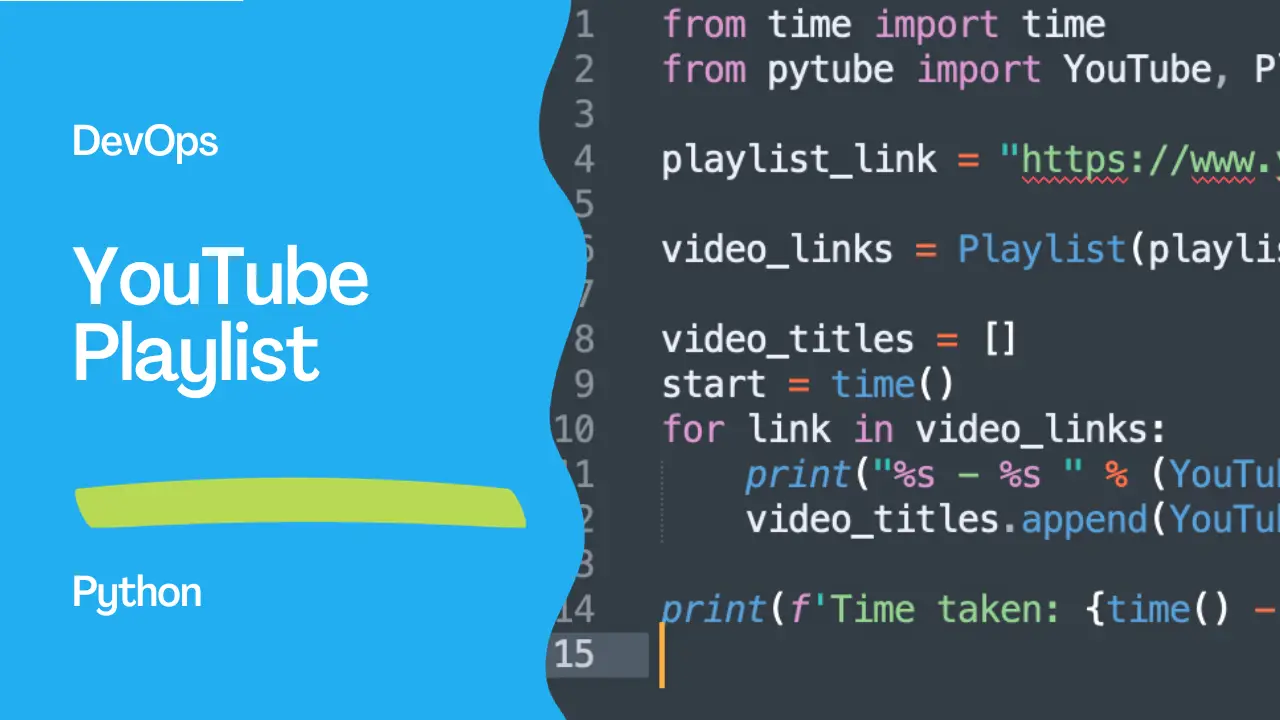
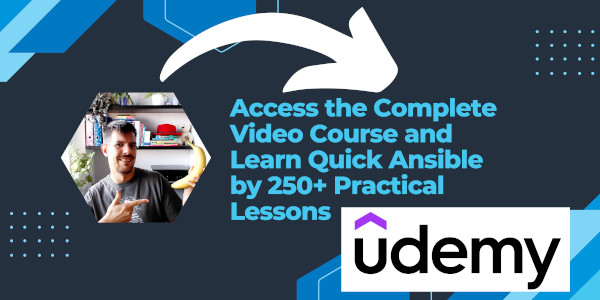
Introduction
YouTube is a vast repository of videos covering a multitude of topics. Sometimes, you may find yourself needing to gather information about multiple videos from a playlist efficiently. In this article, we will explore how to use Python’s Pytube library to automate the retrieval of video details from a YouTube playlist.
Setting Up Pytube
Pytube is a lightweight library that enables you to access YouTube videos and playlists easily. Before you begin, make sure to install the Pytube library by running the following command:
pip install pytube
Now that we have Pytube installed, let’s dive into a simple script that fetches details from a YouTube playlist.
The Script
from time import time
from pytube import YouTube, Playlist
playlist_link = "https://www.youtube.com/playlist?list=PLButRaJV74S6ZPfAnImcpj3fh6LN0obwW"
video_links = Playlist(playlist_link).video_urls
video_titles = []
start = time()
for link in video_links:
video_id = YouTube(link).video_id
video_title = YouTube(link).title
print(f"{video_id} - {video_title}")
video_titles.append(video_title)
print(f'Time taken: {time() - start}')
Understanding the Script:
Importing Libraries:
- We import the
time
module to measure the script’s execution time. - We import the
YouTube
andPlaylist
classes from the Pytube library.
- We import the
Providing Playlist Link:
- Replace the
playlist_link
variable with the URL of the YouTube playlist you want to analyze.
- Replace the
Fetching Video URLs:
- We create a
Playlist
object using the provided link and extract all video URLs from the playlist.
- We create a
Retrieving Video Details:
- For each video URL, we extract the video ID and title using the
YouTube
class from Pytube.
- For each video URL, we extract the video ID and title using the
Printing and Recording Information:
- The script prints the video ID and title for each video in the playlist.
- It also records the video titles in the
video_titles
list.
Measuring Execution Time:
- The script calculates and prints the time taken to fetch information from the entire playlist.
Conclusion
Automating the retrieval of YouTube video details using Pytube simplifies the process, especially when dealing with extensive playlists. This script can be a valuable tool for content creators, researchers, or anyone needing to analyze and organize information from YouTube playlists efficiently. Feel free to customize the script to suit your specific needs. Happy coding!
Subscribe to the YouTube channel, Medium, and Website, X (formerly Twitter) to not miss the next episode of the Ansible Pilot.Academy
Learn the Ansible automation technology with some real-life examples in my
My book Ansible By Examples: 200+ Automation Examples For Linux and Windows System Administrator and DevOps
Donate
Want to keep this project going? Please donate